新用户注册的过程(会话流程)
16lz
2022-05-01
" class="reference-link">#流程分析图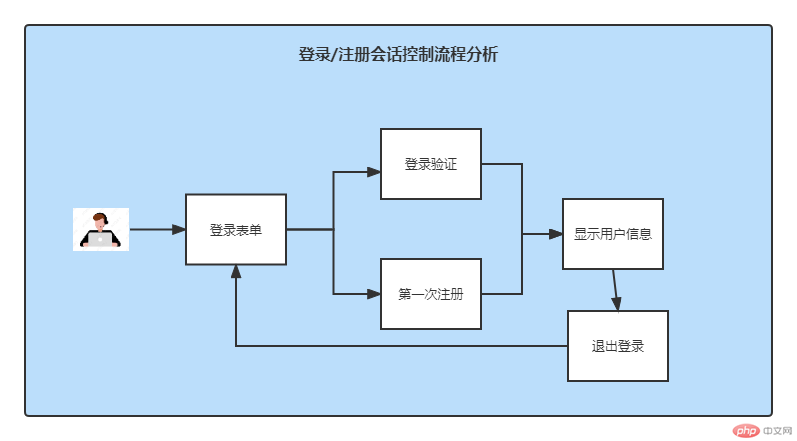
入口文件index.php
<?php
session_start();
// 判断用户是否已经登录?
if (isset($_SESSION['user'])) $user = unserialize($_SESSION['user']);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>session会话处理</title>
</head>
<body>
<nav>
<?php if (isset($user)) : ?>
<a href="" id="logout">退出</a>
<?php else:?>
<a href="login.php">登录</a>
<?php endif ?>
</nav>
<script>
document.querySelector('#logout').addEventListener('click', function(event) {
if (confirm('是否退出?')) {
// 阻止默认的点击事件执行
event.preventDefault();
// Location.assign() 方法会触发窗口加载并显示指定的URL的内容。
location.assign('handle.php?action=logout');
}
});
</script>
</body>
</html>
登录文件login.php
<!DOCTYPE html>
<html lang="en">
<?php
// 判断用户是否已经登录?
if (isset($_SESSION['user'])) echo '<script>alert("不要重复登录");location.href="index.php"</script>';
?>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#form1 {
width: 20em;
border-radius: 0.3em;
box-shadow: 0 0 1em #888;
box-sizing: border-box;
padding: 1em 2em 1em;
margin: 1em auto;
background-color: lightblue;
display: grid;
grid-template-columns: 1fr;
gap: 1em 0;
}
#form1 input {
border-radius: 0.3em;
border: none;
padding-left: 0.3em;
}
#form1 input:focus {
outline: none;
box-shadow: 0 0 5px seagreen;
background-color: hsl(283, 100%, 95%);
border-radius: 0.2em;
transition: 0.5s;
}
#form1 button {
grid-area: auto / 2 / auto;
outline: none;
background-color: royalblue;
border: none;
height: 2em;
color: #fff;
}
#form1 button:hover {
cursor: pointer;
background-color: seagreen;
transition: 0.5s;
}
</style>
</head>
<body>
<form id="form1" action="handle.php?action=login" method="POST" >
<fieldset style="disply:inline-block;background:deepskyblue">
<legend>用户登录</legend>
<p>
<input type="email" name="email" placeholder="user@email.com" required>
</p>
<p>
<input type="password" name="password" placeholder="不少于六位" required>
</p>
<P>
<button>提交</button>
</P>
</fieldset>
<p>
<a href="register.php">如果没有账号,请先注册!</a>
</p>
</form>
</body>
</html>
注册文件register.php
<!DOCTYPE html>
<html lang="en">
<?php
session_start();
// 判断用户是否已经登录?
if (isset($_SESSION['user'])) echo '<script>alert("不要重复登录");locatoin.href="index.php"</script>'
?>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户注册表单</title>
<style>
#form1 {
width: 25em;
border-radius: 0.3em;
box-shadow: 0 0 1em #888;
box-sizing: border-box;
padding: 1em 2em 1em;
margin: 1em auto;
background-color: lightblue;
display: grid;
grid-template-columns: 1fr;
gap: 1em 0;
}
#form1 fieldset {
border: none;
border-radius: 0.3em;
box-shadow: 0 0 1em #888;
box-sizing: border-box;
display: grid;
grid-template-columns: 5em 1fr;
gap: 1em 0;
padding: 1em 2em 1em;
margin: 1em 1em;
}
#form1 fieldset legend
{
grid-area: auto / span 2;
}
#form1 input {
border-radius: 0.3em;
border: none;
padding-left: 0.3em;
}
#form1 input:focus {
outline: none;
box-shadow: 0 0 5px seagreen;
background-color: hsl(283, 100%, 95%);
border-radius: 0.2em;
transition: 0.5s;
}
#btn1 {
grid-area: auto / 2 / auto;
outline: none;
background-color: royalblue;
border: none;
height: 2em;
color: #fff;
}
#btn1:hover {
cursor: pointer;
background-color: seagreen;
transition: 0.5s;
}
</style>
</head>
<body>
<form id="form1" action="handle.php?action=register" method="POST">
<fieldset style="disply:inline-block;background:deepskyblue">
<legend>用户注册</legend>
<label for="name">姓名:</label>
<input type="text" name="name" id="username" placeholder="username" required>
<label for="email">邮箱:</label>
<input type="email" name="email" id="email" placeholder="user@email.com" required>
<label for="password">密码:</label>
<input type="password" name="password" id="psw1" placeholder="不少于六位" required>
<label for="psw1">确认密码:</label>
<input type="password" name="psw1" id="psw2" onblur="check()" placeholder="*两次一致√" required>
<input type="submit" id="btn1" onclick="check()">
</fieldset>
</form>
<script type="text/javascript">
//自定义通过ID获取元素的函数
function $(id){
return document.getElementById(id)
}
function check(){
let check;
if ($('psw1').value != $('psw2').value) {
alert('两次密码不一致,请重新输入');
$('psw1').focus();
check=false;
}else{
alert('两次密码一致');
check=true;
}
return check;
}
</script>
</body>
</html>
注册验证效果图示
控制器文件handle.php
<?php
// 开启会话
// 控制器文件:根据用户的不同请求,执行不同的操作,登录 , 注册, 退出
// 连接数据并获取用户表中的数据
session_start();
$db = new PDO('mysql:dbname=phpedu','root','root');
$stmt = $db->prepare('SELECT * FROM `user`');
$stmt->execute();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
$action = $_GET['action'];
switch (strtolower($action)) {
// 登录
case 'login':
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
extract($_POST);
$result = array_filter($users, function($user) use ($email,$password) {
return $user['email'] === $email && $user['password'] === md5($password);
});
if (count($result) === 1) {
$_SESSION['user'] = serialize(array_pop($result));
// exit、die — 输出一个消息并且退出当前脚本
exit('<script>alert("验证通过");location.href="index.php"</script>');
} else {
exit('<script>alert("邮箱或密码错误");location.href="index.php"</script>');
}
} else {
die('请求错误');
}
break;
// 退出
case 'logout':
if (isset($_SESSION['user'])) {
session_destroy();
exit('<script>alert("退出成功");location.assign("index.php")</script>');
}
break;
// 注册
case 'register':
$name = $_POST['name'];
$email = $_POST['email'];
$password = md5($_POST['password']);
$register_time = time();
$sql = 'INSERT `user` SET `name` = ?, `email` = ?, `password` = ?,`time` = ?';
$stmt = $db->prepare($sql);
$stmt->execute([$name, $email, $password, $register_time]);
if ($stmt->rowCount() === 1) {
echo '<script>alert("注册成功");location.href="index.php"</script>';
} else {
exit('<script>alert("注册失败");location.href="index.php"</script>');
}
break;
}
更多相关文章
- Android(安卓)逆向apk程序的心得
- Android(安卓)渗透测试学习手册(三)Android(安卓)应用的逆向和审计
- 最新res索引讲解(drawable、layout、values等目录的分辨率和layou
- Android之Adapter用法总结
- Windows下的Android(安卓)SDK下载,2.2之前各个版本及Google API,文
- 谈谈android数据存储方式
- Android(安卓)系统权限之SuperSU 模拟器root
- Android(安卓)Building System 总结
- Android虚拟机与Java虚拟机——两种虚拟机的比较